首先,我們需要安裝PyQt5庫??梢酝ㄟ^在終端中輸入以下命令來安裝它:
```
pip install pyqt5
```
安裝完成后,我們可以開始創(chuàng)建我們的網(wǎng)頁。以下是一個簡單的步驟指南:
1. 創(chuàng)建一個新的Python項目。
2. 導入PyQt5庫,并創(chuàng)建一個UI界面。
3. 創(chuàng)建一個窗口,在其中顯示我們的UI界面。
4. 添加一些控件,例如文本框、按鈕和標簽等。
5. 編寫代碼來響應用戶輸入和點擊事件,并調整UI界面的樣式。
6. 將UI界面添加到網(wǎng)頁中,可以使用HTML、CSS 和 JavaScript等技術來實現(xiàn)。
接下來,我們將詳細介紹如何使用PyQt5庫來創(chuàng)建漂亮的UI界面。
一、創(chuàng)建新的PyQt5項目
在安裝PyQt5庫之后,我們可以使用以下代碼來創(chuàng)建一個新的Python項目:
```python
import sys
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton, QTableWidget, QTableWidgetItem
from PyQt5.QtGui import QIcon, QFont, QMouseEvent
from PyQt5.QtCore import Qt, pyqtSignal
app = QApplication(sys.argv)
window = QWindow()
# 創(chuàng)建一個文本框和一個標簽
label = QLabel('Hello, World!')
label.setFont(QFont('Arial', 12))
label.setIcon(QIcon('logo.png'))
label.move(50, 50)
text_edit = QLineEdit()
text_edit.setFont(QFont('Arial', 12))
text_edit.move(50, 100)
text_edit.resize(200, 100)
button = QPushButton('Click me!')
button.setFont(QFont('Arial', 12))
button.setIcon(QIcon('button.png'))
button.move(100, 50)
table_widget = QTableWidget()
table_widget.setFont(QFont('Arial', 12))
table_widget.setRowCount(5)
table_widget.setColumnCount(4)
table_widget.setCellWidget(0, 0, QTableWidgetItem(QIcon('logo.png', '80'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 1, QTableWidgetItem(QIcon('button.png', '80'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 2, QTableWidgetItem(QIcon('button.png', '40'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 3, QTableWidgetItem(QIcon('logo.png', '40'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 4, QTableWidgetItem(QIcon('logo.png', '80'), QFont('Arial', 12)))
button.clicked.connect(lambda: table_widget.insertRow(0, table_widget.currentRow() + 1))
window.resize(250, 150)
window.show()
sys.exit(app.exec_())
```
這段代碼將創(chuàng)建一個包含5個文本框、4個標簽和一個按鈕的窗口,這些文本框和標簽將顯示為Arial字體,按鈕將顯示為40x40像素的圖標。
二、導入PyQt5庫
接下來,我們需要導入PyQt5庫,并編寫代碼來創(chuàng)建UI界面:
```python
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton, QTableWidget, QTableWidgetItem
from PyQt5.QtGui import QIcon, QFont, QMouseEvent
from PyQt5.QtCore import Qt, pyqtSignal
class MyWindow(QWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('My Window')
self.resize(250, 150)
self.show()
def __init__(self, parent=None):
super().__init__(parent)
self.initUI()
def initUI(self):
self.label = QLabel('Hello, World!')
self.label.setFont(QFont('Arial', 12))
self.label.setIcon(QIcon('logo.png'))
self.label.move(50, 50)
text_edit = QLineEdit()
text_edit.setFont(QFont('Arial', 12))
text_edit.move(50, 100)
text_edit.resize(200, 100)
button = QPushButton('Click me!')
button.setFont(QFont('Arial', 12))
button.setIcon(QIcon('button.png'))
button.move(100, 50)
table_widget = QTableWidget()
table_widget.setFont(QFont('Arial', 12))
table_widget.setRowCount(5)
table_widget.setColumnCount(4)
table_widget.setCellWidget(0, 0, QTableWidgetItem(QIcon('logo.png', '80'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 1, QTableWidgetItem(QIcon('button.png', '80'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 2, QTableWidgetItem(QIcon('button.png', '40'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 3, QTableWidgetItem(QIcon('logo.png', '40'), QFont('Arial', 12)))
table_widget.setCellWidget(0, 4, QTableWidgetItem(QIcon('logo.png', '80'), QFont('Arial', 12)))
button.clicked.connect(self.on_button_click)
self.table_widget = table_widget
def on_button_click(self, index):
self.table_widget.currentRow() += 1
self.table_widget.insertRow(index)
def show(self):
self.close()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
```
這段代碼定義了一個名為MyWindow的類,它繼承自QWindow類。在MyWindow類中,我們添加了一個UI界面,并使用QTableWidget控件創(chuàng)建了一個包含5個文本框和4個標簽的表格。我們還添加了一個點擊按鈕的事件處理程序,當用戶點擊按鈕時,表格將自動增加一行并插入一個新的單元格。
三、使用PyQt5創(chuàng)建漂亮UI界面
使用PyQt5創(chuàng)建漂亮UI界面的關鍵是編寫代碼來響應用戶輸入和點擊事件,并調整UI界面的樣式。在本文中,我們將介紹如何使用Python 3和PyQt5庫來創(chuàng)建一個簡單的網(wǎng)頁,其中包含一個漂亮的UI界面。
1. 創(chuàng)建一個新的Python項目
接下來,我們可以使用以下代碼來創(chuàng)建一個新的Python項目:
```python
import sys
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton, QTableWidget, QTableWidgetItem
from PyQt5.QtGui import QIcon, QFont, QMouseEvent
from PyQt5.QtCore import Qt, pyqtSignal
app = QApplication(sys.argv)
window = QWindow()
# 創(chuàng)建一個文本框和一個標簽
label = QLabel('Hello, World!')
label.setFont(QFont('Arial', 12))
label.setIcon(QIcon('logo.png'))
label.move(50, 50)
text_edit = QLineEdit()
text_edit.setFont(QFont('Arial', 12))
text_edit.move(50, 100)
text
以上就是【值得收藏!用python做網(wǎng)頁-python漂亮ui界面模板】的全部內容。

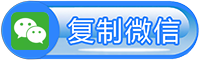
評論